Arrays in JAVA
- oanaunciuleanu
- 24 feb. 2018
- 1 min de citit
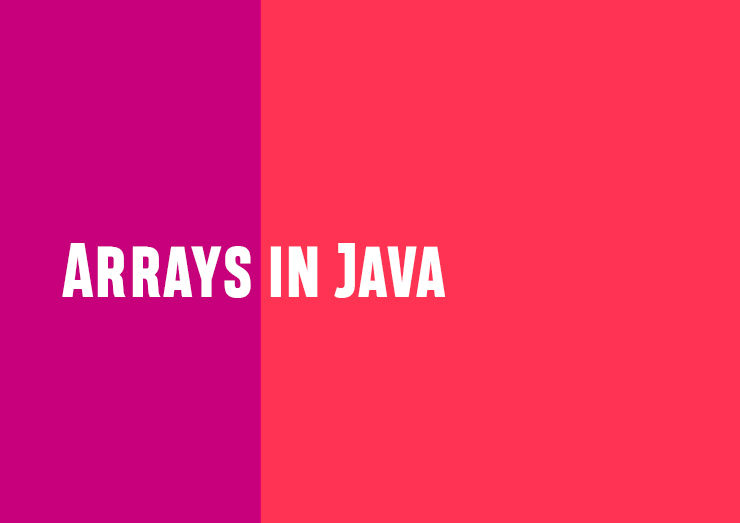
An array stores a fixed-size sequential collection of elements of the same type.
// An array is a succession of values of the same type
// Create an array
String[] iceCreamFlavours = new String[5];
// Initialize the elements in an array
iceCreamFlavours[0] = "Cherry";
iceCreamFlavours[1] = "Vanilla";
iceCreamFlavours[2] = "Chocolate";
iceCreamFlavours[3] = "Mango";
iceCreamFlavours[4] = "Pistachio";
// The index number in an array starts with 0.
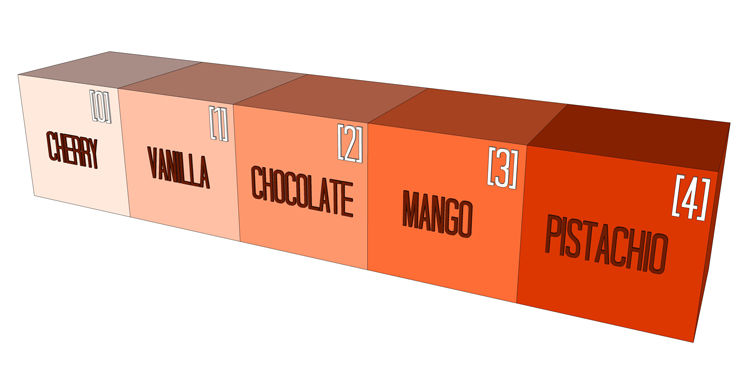
//Access the elements in an array
System.out.println(iceCreamFlavours[0]);
// the value listed is Cherry
// To get the length of an array use .length
System.out.println(iceCreamFlavours.length);
// To access the elements in an array
for (int i = 0; i < iceCreamFlavours.length; i++) {
System.out.println("My favourite ice cream is: " + iceCreamFlavours[i]);
}
// Multidimensional arrays
// Create an array
// The first dimension
String[][] sweets = new String[3][];
// For the second dimension
sweets[0] = new String[4];
sweets[1] = new String[2];
sweets[2] = new String[3];
// Populating the multidimensional array
sweets[0][0] = "pie";
sweets[0][1] = "pudding";
sweets[0][2] = "tarts";
sweets[0][3] = "candies";
sweets[1][0] = "apple strudel";
sweets[1][1] = "baklava";
sweets[2][0] = "parfait";
sweets[2][1] = "caramel sauce";
sweets[2][2] = "frozen yogurt";

// To access the elements in an array for (int i = 0; i < sweets.length; i++) { for (int j = 0; j < sweets[i].length; j++) { System.out.println("I love to eat: " + sweets[i][j]); } }
Comments