Bubble Sort Algorithm in C++
- oanaunciuleanu
- 7 apr. 2020
- 1 min de citit

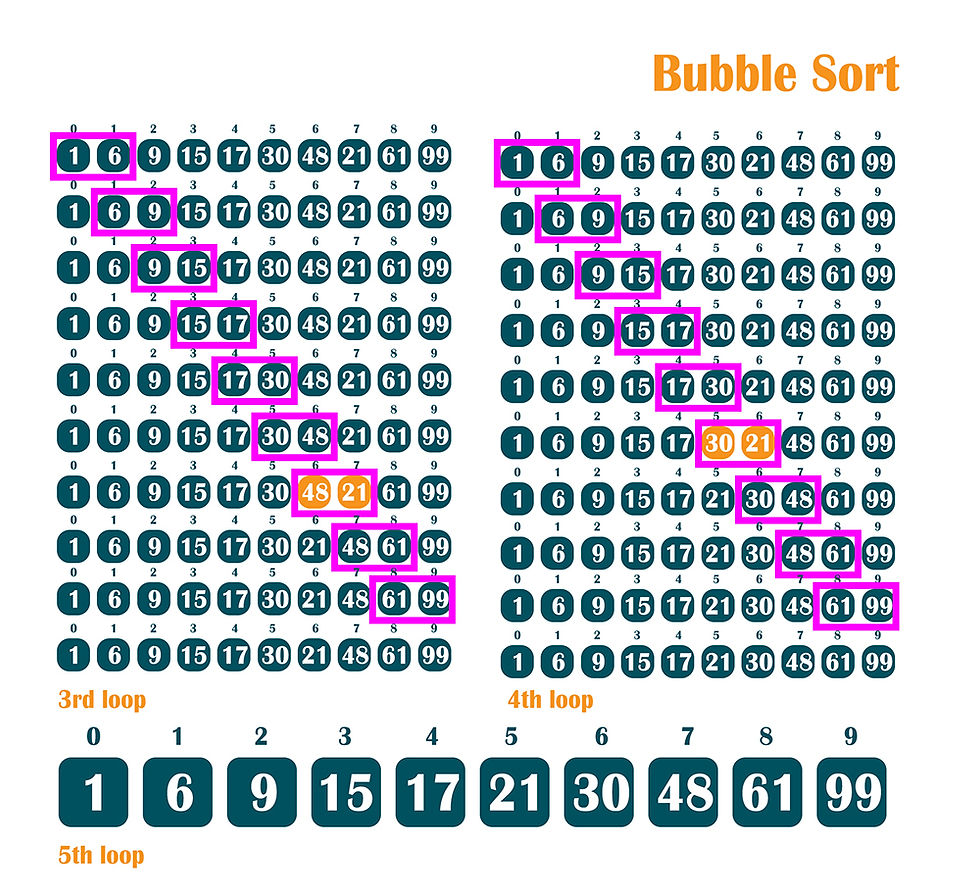
Bubble Sort algorithm is the simplest sorting algorithm. It compares each value with the adjacent value. If the second value is smaller than the first one, then we swap the values. So we compare A[0] with A[1], and if A[0] is greater than A[1] we swap them. This goes on until we finish the array, and then we enter the loop again. This verification is done in a loop, until there is an entire search in the array with no swapping of elements.
// Bubble sort
#include <iostream>
using namespace std;
void bubbleSort(int arrayOfNumbers[], int numberOfElements)
{
for (int i = 0; i < numberOfElements - 1; i++)
{
for (int j = 0; j < numberOfElements - i - 1; j++)
{
if (arrayOfNumbers[j] > arrayOfNumbers[j + 1])
{
int temp = arrayOfNumbers[j];
arrayOfNumbers[j] = arrayOfNumbers[j + 1];
arrayOfNumbers[j+1] = temp;
}
}
}
}
void printElementsOfArray(int arrayOfNumbers[], int numberOfElements)
{
for (int i = 0; i < numberOfElements; i++)
{
cout << arrayOfNumbers[i] << " ";
}
cout << endl;
}
int main()
{
int arrayOfNumbers[] = {1, 15, 9, 6, 17, 99, 48, 30, 61, 21};
int numberOfElements = sizeof(arrayOfNumbers) / sizeof(arrayOfNumbers[0]);
bubbleSort(arrayOfNumbers, numberOfElements);
cout<<"The sorted array is: \n";
printElementsOfArray(arrayOfNumbers, numberOfElements);
return 0;
}
Comments