Caesar Cipher Cryptography Algorithm in C++
- oanaunciuleanu
- 13 apr. 2020
- 2 min de citit
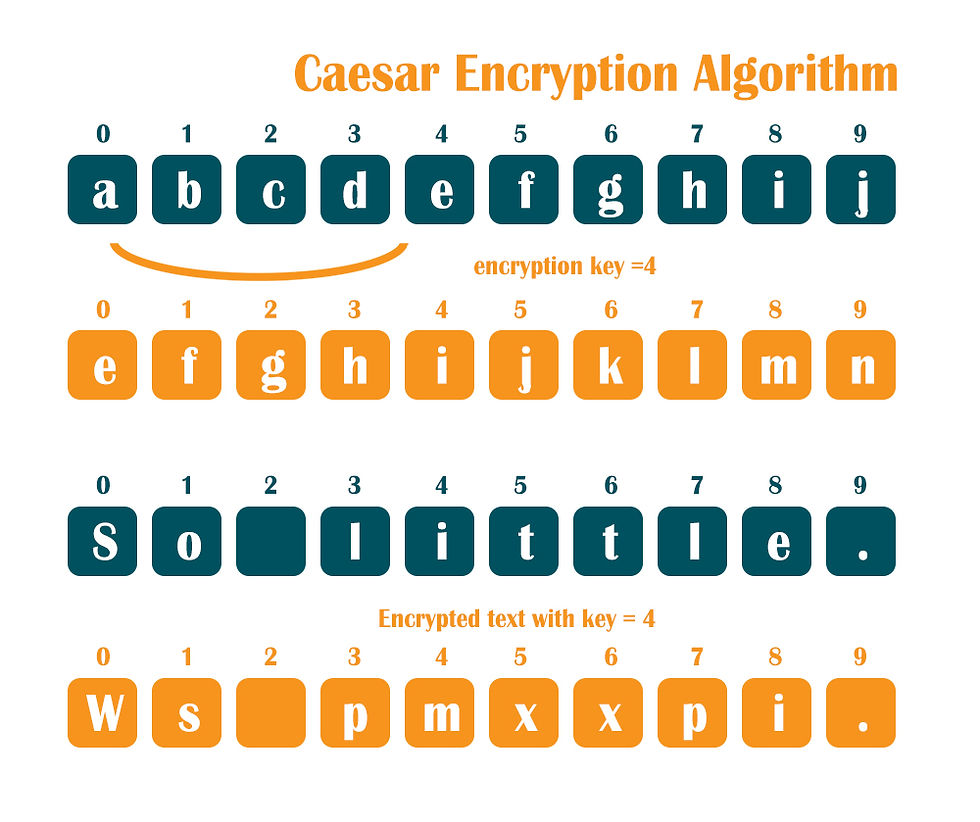
Caesar Cipher is one of the earliest encryption methods, apparently used by Julius Caesar. It is simple to use and implement, and even can be easily broken by hand. It implies that we shift the letters in the alphabet with a certain key. If the key is 2, then A becomes C, C becomes D and so one.
// Caesar Algorithm
#include <iostream>
using namespace std;
string encriptCaesar(string originalText, int key)
{
string result = "";
// I search the text one letter at a time
for (int i = 0; i < originalText.length(); i++)
{
// ASCII decimal: (space) = 32
if (originalText[i] == 32)
{
result += " ";
}
// ASCII decimal: . = 46
else if(originalText[i] == 46)
{
result += ".";
}
// ASCII decimal: , = 44
else if(originalText[i] == 44)
{
result += ",";
}
// For UPPER case letters
// ASCII decimal: A = 65, Z = 90, 26 characters
else if (isupper(originalText[i]))
{
result += char(int(originalText[i] + key - 65) % 26 + 65);
}
// ASCII decimal: a = 97, z = 122, 26 characters
// For lower letters
else
{
result += char(int(originalText[i] + key - 97) % 26 + 97);
}
}
return result;
}
int main()
{
string originalText = "But soft what light through yonder window breaks.";
int key = 12;
int decriptKey = 26 - key;
cout << "Original text: " << originalText << endl;
cout << "The key is: " << key << endl;
string encryptedMessage = encriptCaesar(originalText, key);
cout << "The encrypted message: " << encryptedMessage<< endl;
string decryptedMessage = encriptCaesar(encryptedMessage, decriptKey);
cout << "The decrypted message: " << decryptedMessage << endl;
return 0;
}
Result:
Original text: But soft what light through yonder window breaks.
The key is: 12
The encrypted message: Ngf earf itmf xustf ftdagst kazpqd iuzpai ndqmwe.
The decrypted message: But soft what light through yonder window breaks.
Comentarios