Linear Search in C++
- oanaunciuleanu
- 3 apr. 2020
- 1 min de citit
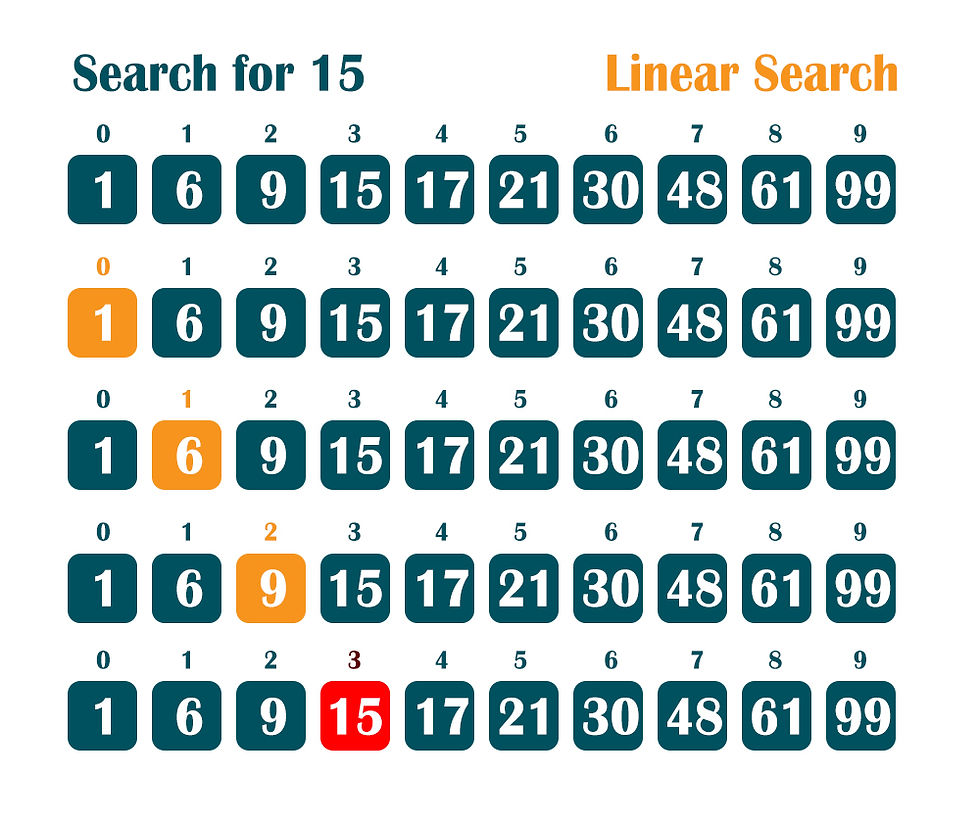
Linear Search is a simple way to search for a value in an array. It looks through the values in the array one by one and compares them to the searched value. It is not used very often for large sets because other search algorithms perform better.
// Linear Search
#include <iostream>
using namespace std;
int linearSearch(int arrayOfNumbers[], int searchForNumber, int numberOfElements)
{
for (int i = 0; i < numberOfElements; i++)
{
if (arrayOfNumbers[i] == searchForNumber)
{
printf("The number %d is found at index %d", searchForNumber, i);
return i;
}
}
printf("The number %d was not found in the array", searchForNumber);
return -1;
}
int main(void)
{
int arrayOfNumbers[] = {1, 6, 9, 15, 17, 21, 30, 48, 61, 99};
int searchForNumber = 15;
int numberOfElements = sizeof(arrayOfNumbers) / sizeof(arrayOfNumbers[0]);
int result = linearSearch(arrayOfNumbers, searchForNumber, numberOfElements );
}
Comments